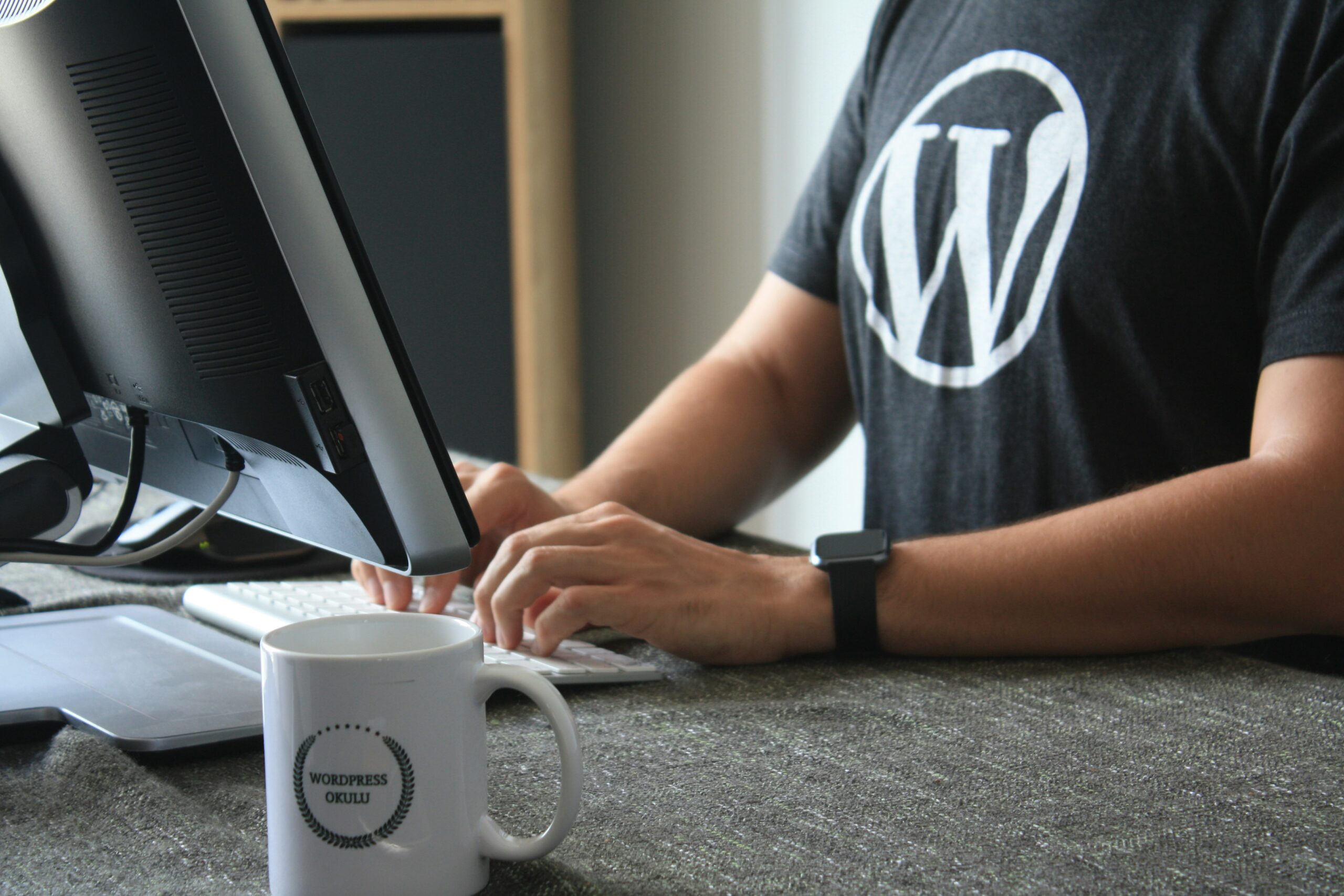
How to Create a Simple WordPress Plugin to Extend Custom Options for a Better WordPress Website
Introduction to WordPress Plugin Development
WordPress plugin development serves as an indispensable skill for anyone looking to amplify the functionality and user experience of their website. A plugin in WordPress is a piece of software that can be added to a WordPress site to enhance its capabilities. Whether you wish to include additional features, optimize site performance, or broaden customization options, a plugin offers an efficient solution to these needs.
Before diving into the creation process, it’s crucial to familiarize yourself with some prerequisites. A working knowledge of PHP, the scripting language at the heart of WordPress, is essential. Additionally, understanding WordPress coding standards will ensure your plugin is reliable and maintainable. You will also need access to a WordPress installation, either locally or on a live server, to test and refine your plugin as you develop it.
Creating custom plugins provides significant advantages over solely relying on existing plugins or themes. These bespoke solutions allow you to address specific requirements, which may not be possible through general-purpose plugins. For example, you may want to include features unique to your business model or industry, achieving a tailored user experience that sets your site apart from competitors. Furthermore, customized plugins offer improved site performance as they can be designed to fulfill exact needs without extraneous code, unlike more generalized plugins.
The flexibility and control that WordPress plugins provide cannot be overstated. Whether you are a novice or an experienced developer, diving into plugin development can open new avenues for website customization and functionality enhancement. As we proceed through this guide, you will gain insights into the step-by-step process of WordPress plugin development, starting from setting up your development environment to deploying your bespoke solutions effectively.
Step-by-Step Guide to Creating a Simple WordPress Plugin
Creating a simple WordPress plugin is a practical way to extend the functionality of a WordPress website. This step-by-step guide will navigate through the essential processes needed to develop a basic plugin, from setting up a development environment to implementing and testing custom functionalities.
Setting Up the Development Environment
Firstly, ensure that you have a local development environment set up with the latest version of WordPress installed. Common tools for local development include XAMPP, MAMP, or a virtual environment like Vagrant. Install a code editor such as Visual Studio Code or Sublime Text, which will be used to write your plugin’s code.
Creating the Main Plugin File
Navigate to the /wp-content/plugins/
directory within your WordPress installation and create a new folder for your plugin. For instance, name it simple-custom-plugin
. Inside this directory, create the main plugin PHP file, e.g., simple-custom-plugin.php
.
Defining Plugin Header Information
At the top of your simple-custom-plugin.php
file, include the necessary plugin header information within PHP tags to define your plugin:
<?php
/*
Plugin Name: Simple Custom Plugin
Plugin URI: https://example.com/simple-custom-plugin
Description: A simple plugin to demonstrate custom options.
Version: 1.0.0
Author: Your Name
Author URI: https://example.com
License: GPL2
*/
?>
Writing the Core Functions
Now, it’s time to define the core functionalities of your plugin. This typically includes creating functions that execute the desired features of your plugin. For example:
function simple_custom_function() {
// Your custom code here
}
Hook this function into WordPress’s actions or filters:
add_action('init', 'simple_custom_function');
Adding Custom Options
To add custom options, utilize the WordPress Settings API to create settings fields within the admin interface. Begin by creating a settings page:
function simple_custom_menu() {
add_menu_page('Simple Custom Settings', 'Custom Settings', 'manage_options', 'simple-custom-settings', 'simple_custom_settings_page');
}
add_action('admin_menu', 'simple_custom_menu');
function simple_custom_settings_page() {
?>
<div class="wrap">
</div>
<?php
}
Leveraging WordPress Hooks and Filters
Integrate the custom options. Hooks, such as admin_init
and filters, allow for seamless integration:
function simple_custom_register_settings() {
register_setting('simple_custom_settings_group', 'simple_custom_option');
add_settings_section('simple_custom_section', 'Custom Options', null, 'simple-custom-settings');
add_settings_field('custom_option', 'Custom Option', 'custom_option_callback', 'simple-custom-settings', 'simple_custom_section');
}
add_action('admin_init', 'simple_custom_register_settings');
function custom_option_callback() {
?>
<input type="text" name="simple_custom_option" value="<?php echo get_option('simple_custom_option'); ?>">
<?php
}
Testing and Debugging
After writing your plugin, thoroughly test it within your local environment to ensure it works as intended. Use the WordPress debug mode by setting define('WP_DEBUG', true);
in wp-config.php
to identify and resolve any errors. Additionally, employ tools like Query Monitor
for an advanced debugging experience.
Best Practices
Follow best practices such as sanitizing user inputs, escaping outputs, adhering to the WordPress Coding Standards, and ensuring your plugin is compatible with the latest WordPress version. This will enhance the security and reliability of your plugin, making it a valuable addition to any WordPress site.
By following these steps, one can create a simple yet effective WordPress plugin that extends custom options, empowering users to tailor their websites to better meet their needs.