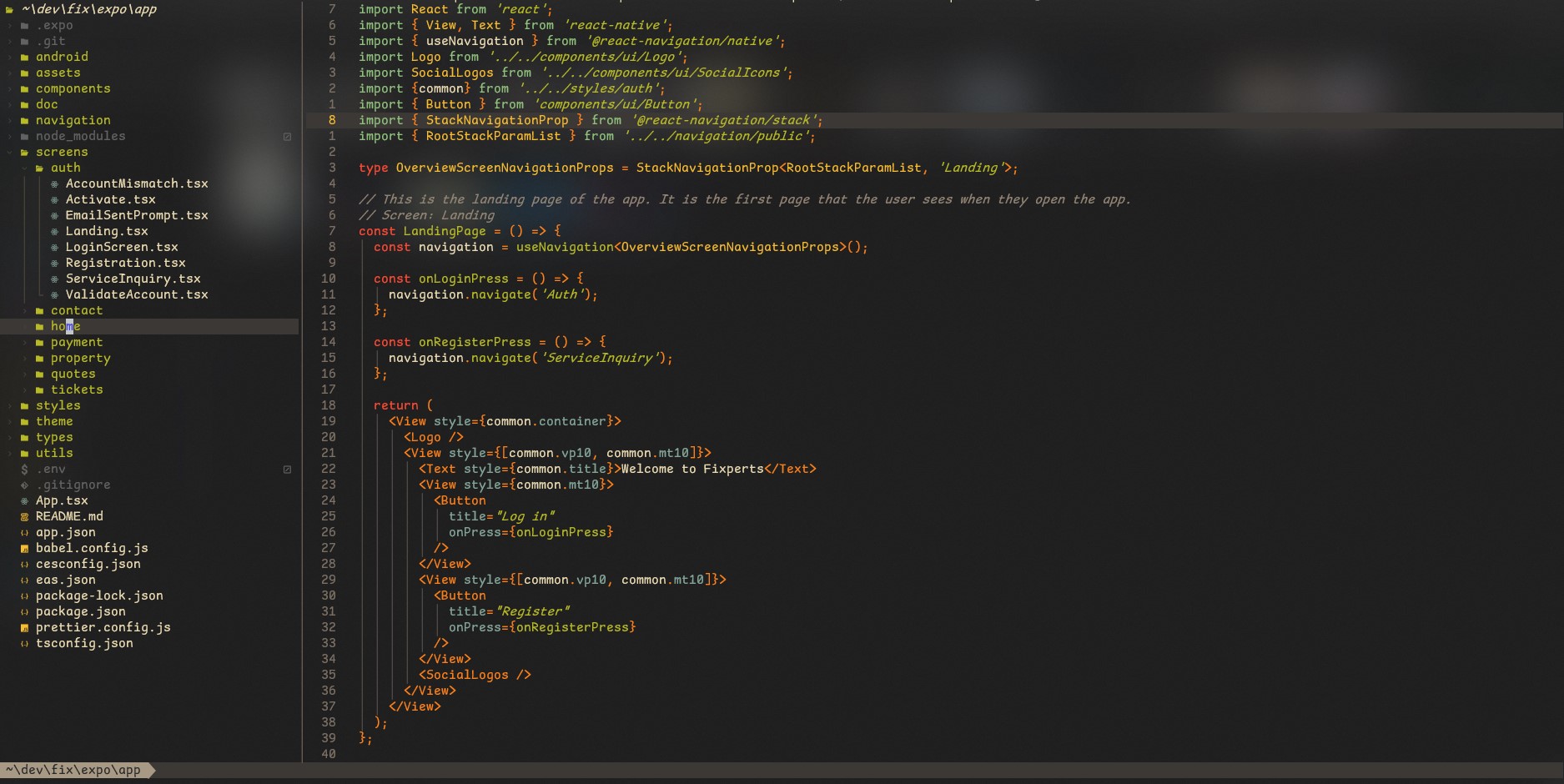
How to Create a React Native Mobile App Using WordPress REST API
Introduction
React Native has become a popular choice for building mobile applications due to its ability to create cross-platform apps with a single codebase. If you’re looking to create a mobile app using React Native and integrate it with WordPress, the WordPress REST API provides a powerful solution. In this guide, we will walk you through the steps to create a React Native mobile app using the WordPress REST API.
Step 1: Set Up Your Development Environment
Before we start building our React Native app, we need to set up our development environment. Make sure you have Node.js and npm installed on your machine. You can check if they are installed by running the following commands in your terminal:
node -v
npm -v
If you don’t have Node.js and npm installed, you can download and install them from the official Node.js website.
Step 2: Create a New React Native Project
Once your development environment is set up, you can create a new React Native project by running the following command in your terminal:
npx react-native init MyApp
Replace “MyApp” with the desired name of your project. This command will create a new directory with the specified name and set up a basic React Native project structure.
Step 3: Install Dependencies
In order to make API requests to your WordPress site, we need to install some dependencies. Navigate to your project directory and run the following command:
cd MyApp
npm install axios
This will install the Axios library, which we will use to make HTTP requests to the WordPress REST API.
Step 4: Set Up WordPress REST API
To enable the WordPress REST API on your WordPress site, you need to install and activate the WP REST API plugin. Once activated, the API will be available at https://site.com/wp-json/wp/v2/
.
Step 5: Fetch Data from WordPress
Now that we have our project set up and the WordPress REST API enabled, we can start fetching data from our WordPress site. Open the App.js
file in your project directory and replace the existing code with the following:
import React, { useEffect, useState } from 'react';
import { View, Text } from 'react-native';
import axios from 'axios';
const App = () => {
const [posts, setPosts] = useState([]);
useEffect(() => {
const fetchPosts = async () => {
try {
const response = await axios.get('https://site.com/wp-json/wp/v2/posts');
setPosts(response.data);
} catch (error) {
console.error(error);
}
};
fetchPosts();
}, []);
return (
<View>
{posts.map((post) => (
<Text key={post.id}>{post.title.rendered}</Text>
))}
</View>
);
};
export default App;
Replace https://site.com
with the URL of your WordPress site. This code fetches the latest posts from your WordPress site and displays their titles in a React Native view.
Step 6: Run Your App
To run your React Native app on a simulator or a physical device, use the following command:
npx react-native run-ios
This command will build your app and launch it on the iOS simulator. If you want to run your app on an Android emulator, use the following command instead:
npx react-native run-android
Congratulations! You have successfully created a React Native mobile app using the WordPress REST API. You can now further customize your app by fetching different types of data from your WordPress site and integrating additional features.
Conclusion
Integrating a React Native mobile app with the WordPress REST API allows you to leverage the power of WordPress and create a seamless user experience. By following the steps outlined in this guide, you can create a mobile app that fetches data from your WordPress site and displays it in a React Native view. Happy coding!