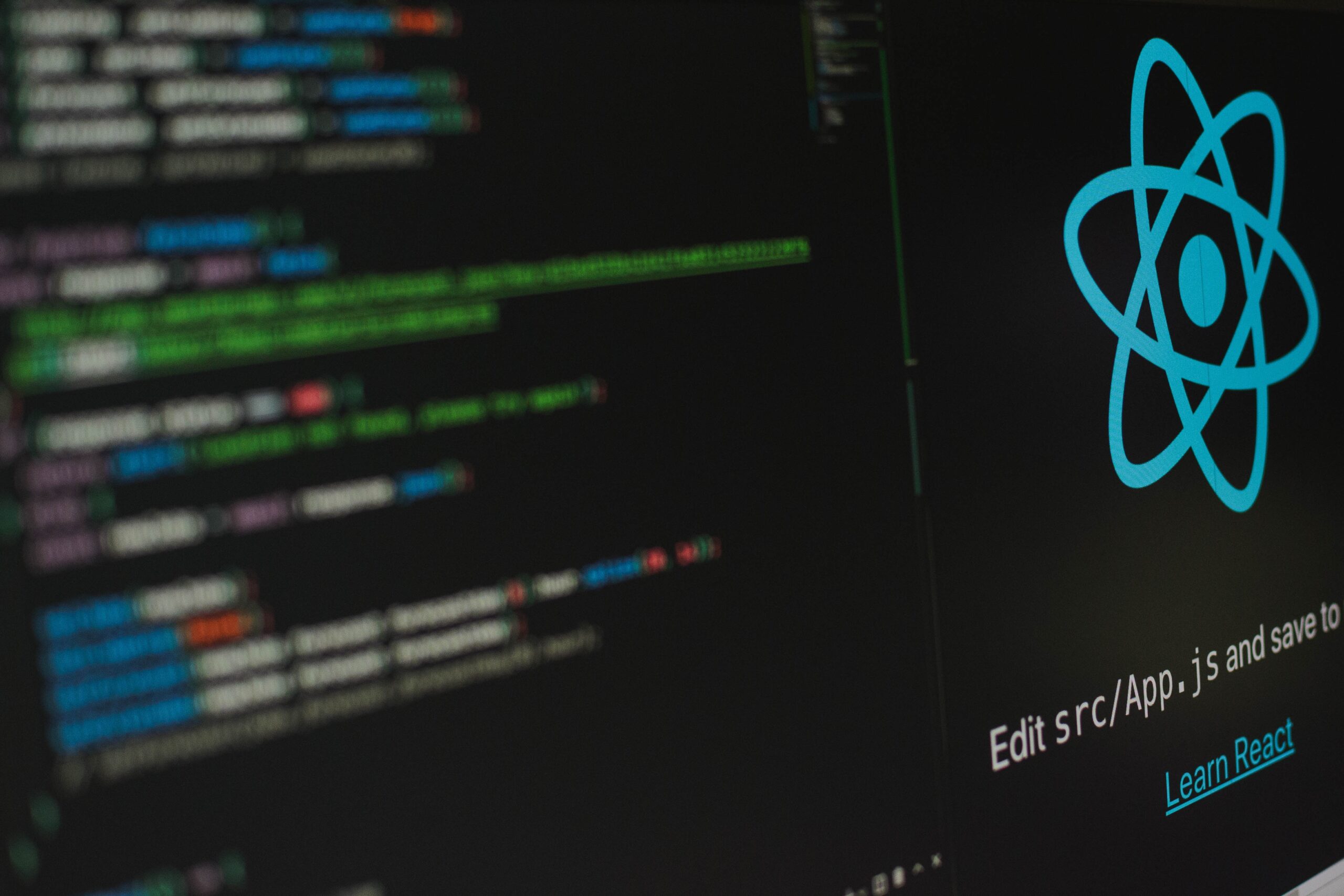
Creating Your First React Component with JSX and Tailwind: A Beginners Guide
Introduction
Are you ready to dive into the exciting world of React? In this blog post, we’ll walk you through the process of creating your first React component using JSX and Tailwind. Whether you’re a seasoned developer looking to learn a new framework or a beginner just starting out, this guide will provide you with step-by-step instructions to get you up and running.
Getting Started
Before we start building our first React component, let’s make sure we have everything we need. First, ensure that you have Node.js installed on your computer. You can download it from the official Node.js website. Once you have Node.js installed, open your terminal and navigate to the directory where you want to create your React project.
Next, let’s set up our React project. Open your terminal and run the following command:
npx create-react-app my-app
This command will create a new directory called ‘my-app’ with all the necessary files and dependencies to start our React project.
Creating the Component
Now that we have our React project set up, let’s start creating our first component. Open the ‘src’ folder in your project directory and create a new file called ‘BookList.js’.
In the ‘BookList.js’ file, let’s import React and define our component. Add the following code:
import React from 'react';
const BookList = () => {
const books = ['Book 1', 'Book 2', 'Book 3'];
return (
<div className='book-list'>
<h2>My Book List</h2>
<ul>
{books.map(book => ({book} ))}
</ul>
</div>
);
};
export default BookList;
In this code, we define a constant ‘books’ that contains an array of book names. We use the map function to iterate over the array and render each book name as a list item within an unordered list.
Using the Component
Now that we have our component created, let’s use it in our main App component. Open the ‘App.js’ file in the ‘src’ folder and replace the existing code with the following:
import React from 'react';
import BookList from './BookList';
const App = () => {
return (
<div className='app'>
<h1>My React App</h1>
<BookList />
</div>
);
};
export default App;
In this code, we import our ‘BookList’ component and render it within our main ‘App’ component. When you save these changes and go back to your browser, you should see the ‘My Book List’ heading and the list of book names rendered on the screen.
Conclusion
Congratulations! You have successfully created your first React component using JSX and Tailwind. This is just the beginning of your journey with React, and there’s so much more to explore. Keep practicing, experimenting, and building amazing things with React. Happy coding!