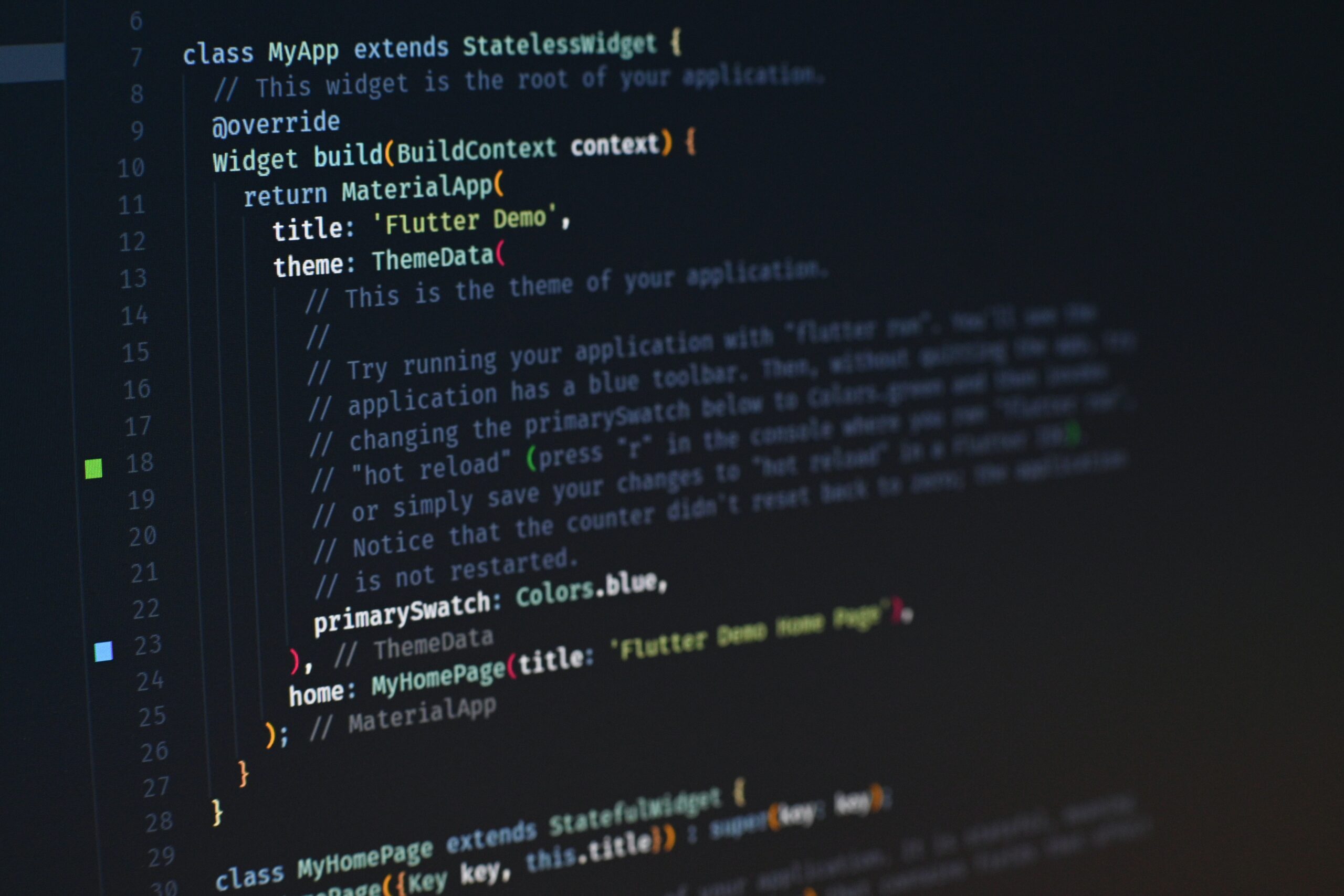
Create Amazing Mobile Apps with Flutter and WebView Integration
Introduction:
In today’s digital age, having a mobile app for your website can greatly enhance user experience and engagement. Flutter, a popular UI toolkit, allows developers to create beautiful and high-performance mobile apps for both Android and iOS platforms. In this blog post, we will guide you through the process of integrating a WebView into your Flutter app, making it easy for users to access your website’s content on their mobile devices.
Section 1: Getting Started with Flutter and WebView:
To begin, make sure you have Flutter and the necessary development tools installed on your machine. Once everything is set up, open your Flutter project and add the ‘webview_flutter’ package to your pubspec.yaml file. This package provides a WebView widget that we will use to load and display web content within our app.
Next, run ‘flutter pub get’ in your terminal to fetch the package and its dependencies. This will ensure that you have the latest version of the ‘webview_flutter’ package available for use in your project.
Section 2: Implementing WebView in Flutter:
Now that you have the ‘webview_flutter’ package installed, it’s time to integrate the WebView into your app. Start by creating a new Dart file, let’s call it ‘webview_screen.dart’, and import the necessary dependencies:
import 'package:flutter/material.dart';
import 'package:webview_flutter/webview_flutter.dart';
Next, create a new StatefulWidget called ‘WebViewScreen’, which will be responsible for displaying the WebView. In the ‘build’ method of the state class, instantiate a WebView widget and pass in the URL of your website:
class WebViewScreen extends StatefulWidget { final String url;
WebViewScreen({this.url});
@override _WebViewScreenState createState() => _WebViewScreenState(); }
class _WebViewScreenState extends State { @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text(‘My Website’), ), body: WebView( initialUrl: widget.url, ), ); } }
Section 3: Navigation and WebView Controls:
With the WebView integrated into your app, you can now add navigation and control options to enhance the user experience. For example, you can add a progress indicator to show the loading status of the web page, or include a back button to allow users to navigate back to the previous page.
Additionally, you can handle errors and display error messages when a web page fails to load. This can help in providing better user feedback and troubleshooting any issues that may arise during the usage of your app.
Conclusion:
By integrating a WebView into your Flutter app, you can provide your users with a seamless browsing experience, allowing them to access your website’s content on their mobile devices. Flutter’s easy-to-use framework and the ‘webview_flutter’ package make it simple to create amazing mobile apps with webview integration. So why wait? Start building your Flutter app today and bring your website to life on mobile platforms!