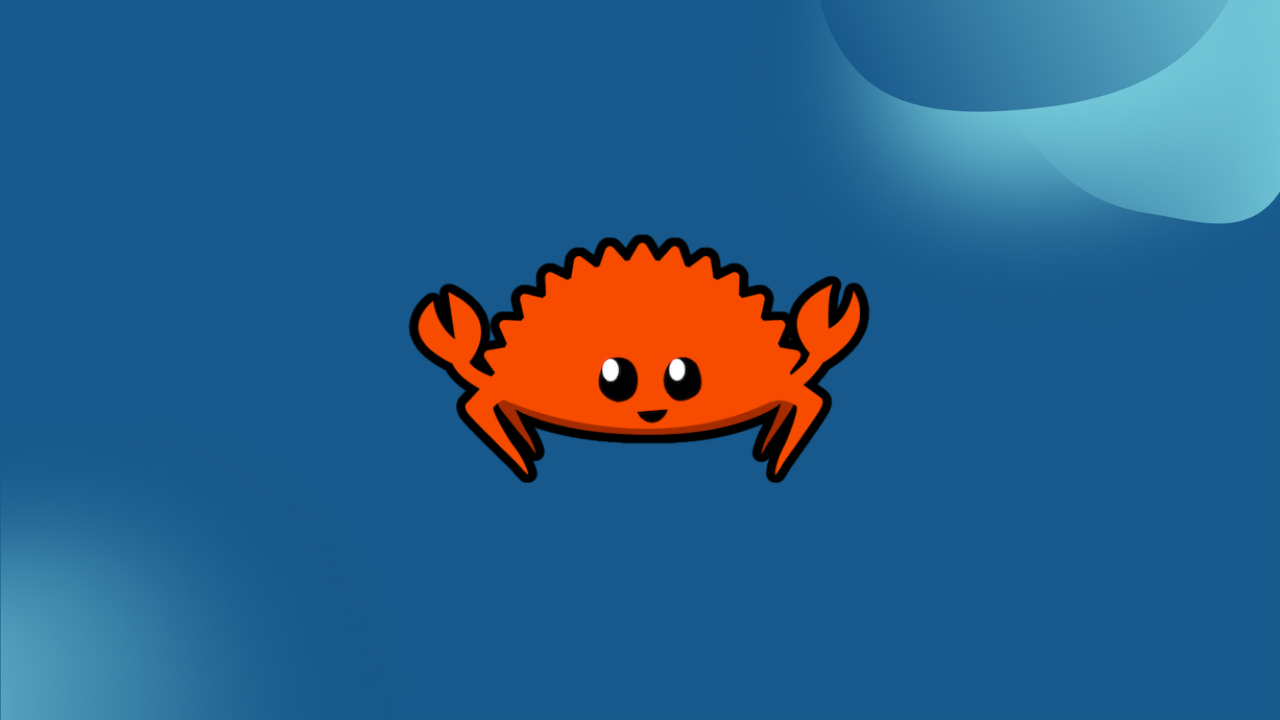
Building a Rust Web Application with Actix and Tokio
Rust is a powerful programming language that has gained popularity for its focus on performance, safety, and concurrency. If you're looking to build a web application in Rust, Actix and Tokio are two frameworks that can help you get started. In this guide, we'll walk you through the process of building a Rust web application using Actix and Tokio.
1. Setting up your Rust environment
Before we dive into building our web application, let's make sure we have a proper Rust environment set up. Start by installing Rust and Cargo, the package manager for Rust. You can find detailed installation instructions on the official Rust website.
2. Creating a new Rust project
Once your Rust environment is set up, it's time to create a new project. Open your terminal and navigate to the directory where you want to create your project. Run the following command to create a new Rust project:
cargo new my_web_app
This will create a new directory called "my_web_app" with the basic structure of a Rust project.
3. Adding Actix and Tokio dependencies
In order to use Actix and Tokio in our project, we need to add them as dependencies in our Cargo.toml file. Open the Cargo.toml file in your project directory and add the following lines:
[dependencies]
actix-web = "3.3"
tokio = { version = "1", features = ["full"] }
This will add Actix and Tokio as dependencies to our project.
4. Writing the web application code
Now that we have our project set up and the dependencies added, let's start writing the code for our web application. Open the src/main.rs file in your project directory and replace the existing code with the following:
use actix_web::{web, App, HttpResponse, HttpServer};
use tokio::time::{sleep, Duration};
async fn index() -> HttpResponse {
// Simulate some async work
sleep(Duration::from_secs(2)).await;
HttpResponse::Ok().body("Hello, Actix and Tokio!")
}
#[actix_web::main]
async fn main() -> std::io::Result<()> {
HttpServer::new(|| {
App::new()
.route("/", web::get().to(index))
})
.bind("127.0.0.1:8080")?
.run()
.await
}
This code sets up a basic Actix web server with a single route ("/") that returns a "Hello, Actix and Tokio!" message after a 2-second delay. Feel free to modify this code to suit your needs.
5. Running the web application
With the code in place, it's time to run our web application. Open your terminal, navigate to your project directory, and run the following command:
cargo run
This will compile and run your Rust web application. You should see output indicating that the server is running on "127.0.0.1:8080". Open your web browser and navigate to "http://127.0.0.1:8080" to see your web application in action.
6. Further exploration
Congratulations! You've successfully built a Rust web application using Actix and Tokio. This is just the tip of the iceberg when it comes to what you can do with Actix and Tokio. Take some time to explore their documentation and experiment with building more complex web applications.
Remember, Actix and Tokio are powerful tools for building high-performance, concurrent web applications in Rust. With their combined capabilities, you can create web applications that handle thousands of requests per second with ease.
Happy coding!